Reverse - CSaw2023
Intro - Baby's First
Author : ElykDeer
Description
Reversing means reading code. Read this file, and find the flag !
Solution
This channel is very easy and beginner friendly. Just as the description suggests, all we have to do is read the file.
#!/usr/bin/env python3
# Reversing is hard. But....not always.
#
# Usually, you won't have access to source.
# Usually, these days, programmers are also smart enough not to include sensitive data in what they send to customers....
#
# But not always....
if input("What's the password? ") == "csawctf{w3_411_star7_5om3wher3}":
print("Correct! Congrats! It gets much harder from here.")
else:
print("Trying reading the code...")
# Notes for beginners:
#
# This is Python file. You can read about Python online, but it's a relatively simple programming language.
# You can run this from the terminal using the command `python3 babysfirst.py`, but I'll direct you to the internet again
# for how to use the terminal to accomplish that.
#
# Being able to run this file is not required to find the flag.
#
# You don't need to know Python to read this code, to guess what it does, or to solve the challenge.
Now if we look at the code closer, we can see that the program is taking user input and comparing it to the flag.
if input("What's the password? ") == "csawctf{w3_411_star7_5om3wher3}":
Flag
csawctf{w3_411_star7_5om3wher3}
Intro - Baby's Third
Author : ElykDeer
Description
Babies can't count, but they can do binaries?
(Where did Baby Two go ?)
Solution
I think this is not the intended solution as it seems to not be relevant to the description or the contents of the readme.txt file, but if we use the strings command on the file. If we filter for the flag, we end up getting it just by that.
strings babythird | grep csawctf{.*}
Explanation: We use the strings command to get all the sequences of printable characters in the babythird file. Then we are pining that to grep, to look for any sequence that starts with csawctf{, ends with }, and has any characters in between (hence the .*).
Flag
csawctf{w3_411_star7_5om3wher3}
Reverse - Rebug 1
Description
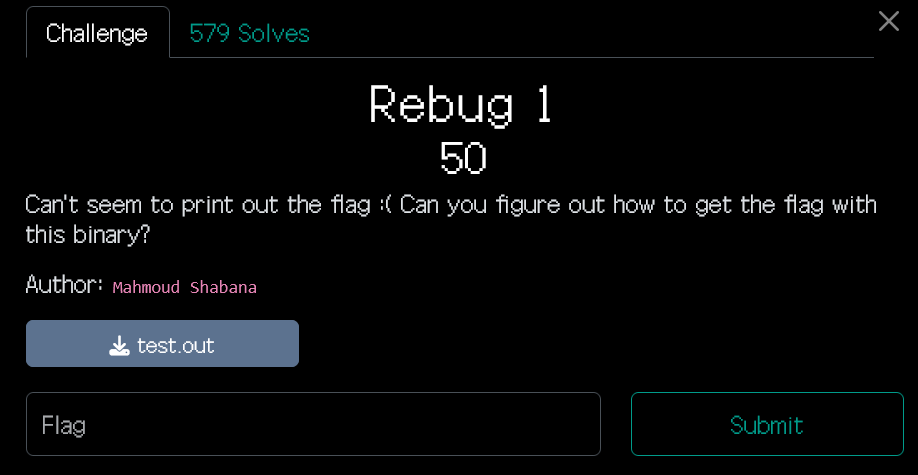
Solution
Open the binary file in Ghidra.
Look at the exports folder to see the exported functions from the binary. There you will find the main function.
Once you have the main function in the decompiler window, you will see the string of the terminal ouput. This will prove that you are in the main function.
You will see that there is for loop incrementing with the number of char variables inside the user's input.
The variable is then checked in a conditional to see if it matches the hex value "0xc", which is 12 in decimal.
Once you submit a 12-character long input, it will execute the correct code block in the if-else statement. This will return the flag, which is the md5 encoding of the string "12".
Reverse - Rebug 2
Description
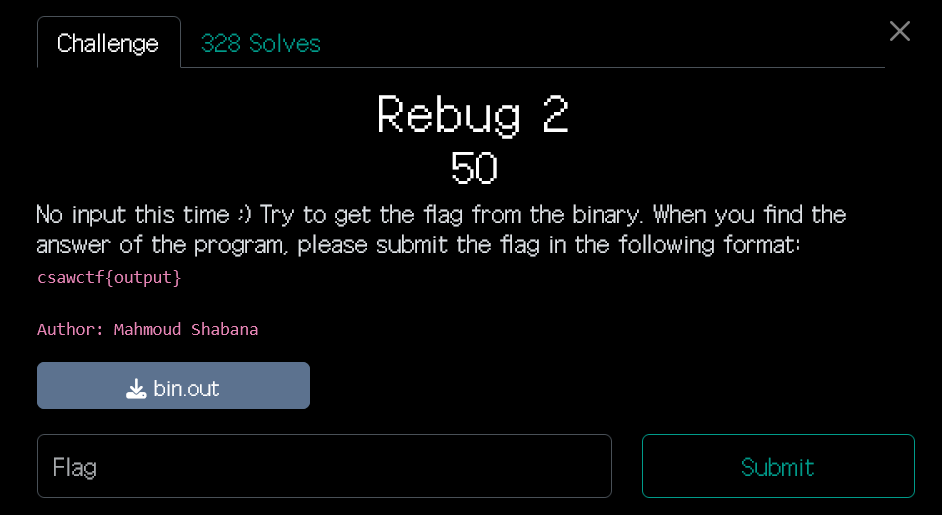
Solution
Given the binary, we need to inspect the decompiled ELF file in ghidra.
We can see that there is not user input being accepted, but rather a hardcoded string is parsed through.
It looks like ever second character is passed to a function called "printbinchar".
Looking at printbinchar(), we see that each character is converted into a binary.
The characters that are passed through would be "AY7Cw" and the binary of each value would be:
A = 01000001 Y = 01011001 7 = 00110111 C = 01000011 w = 01110111
For each character, the "xoring" function is called. The binary for each character is passed through this function.
In the xoring() function, the binary is split down the middle into two 4-bit arrays. Then the xor operation is executed between the two arrays. The result for each character would be:
A = XOR(0100, 0001) = 0101 Y = XOR(0101, 1001) = 1100 7 = XOR(0011, 0111) = 0100 C = XOR(0100, 0011) = 0111 w = XOR(0111, 0111) = 0000
with each XOR result concatenated in order, this returns the flag: csawctf{01011100010001110000}
Other way :
Put a breakpoint on the ret instruction of the xoring function with cutter
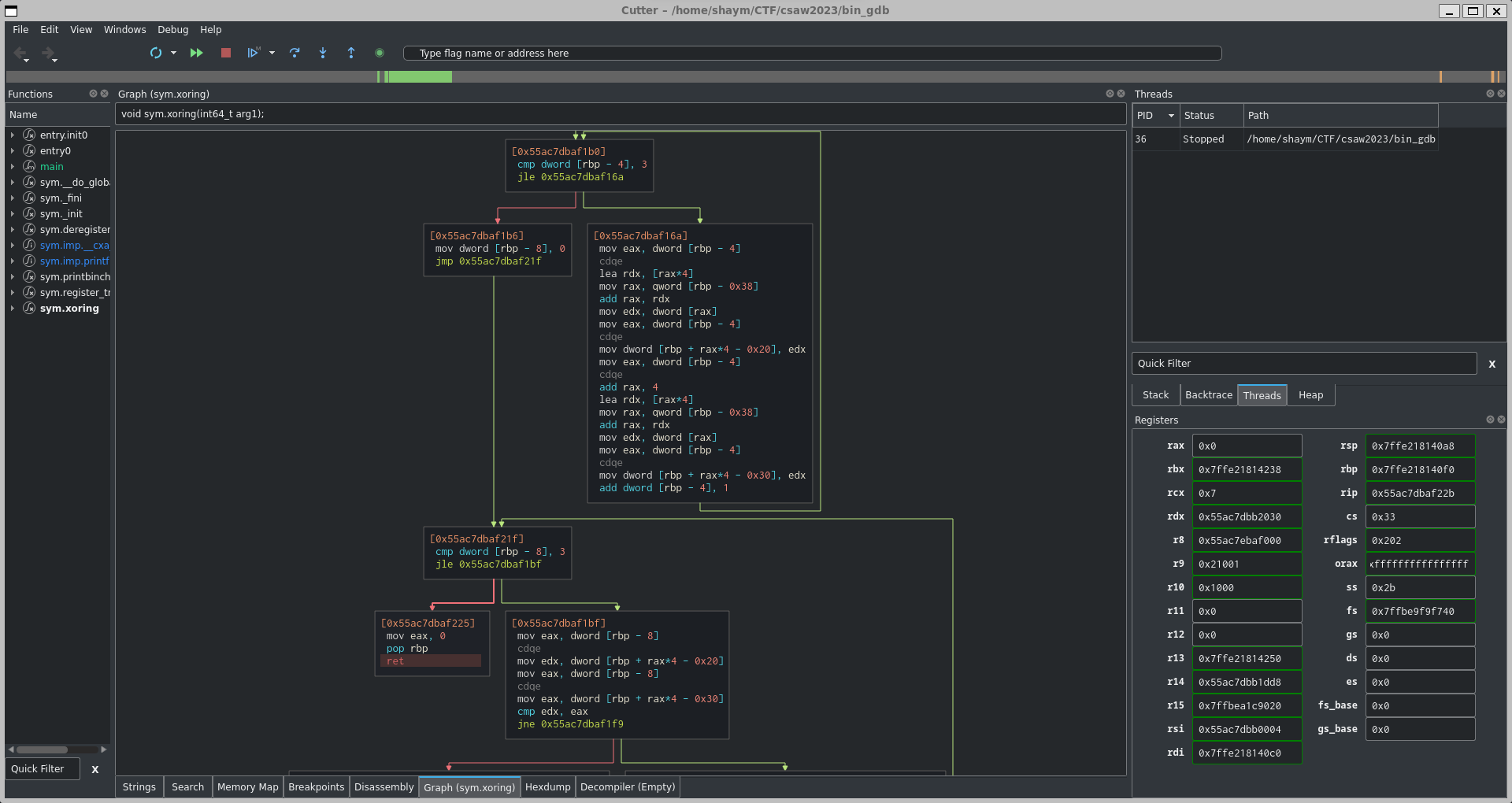
Using cutter put a bp on the ret func of xoring
Run decompiler get the register of flag → rdx
Show in Hexdump
4x clic on continu and get the entier value → 01011100010001110000
csawctf{01011100010001110000}
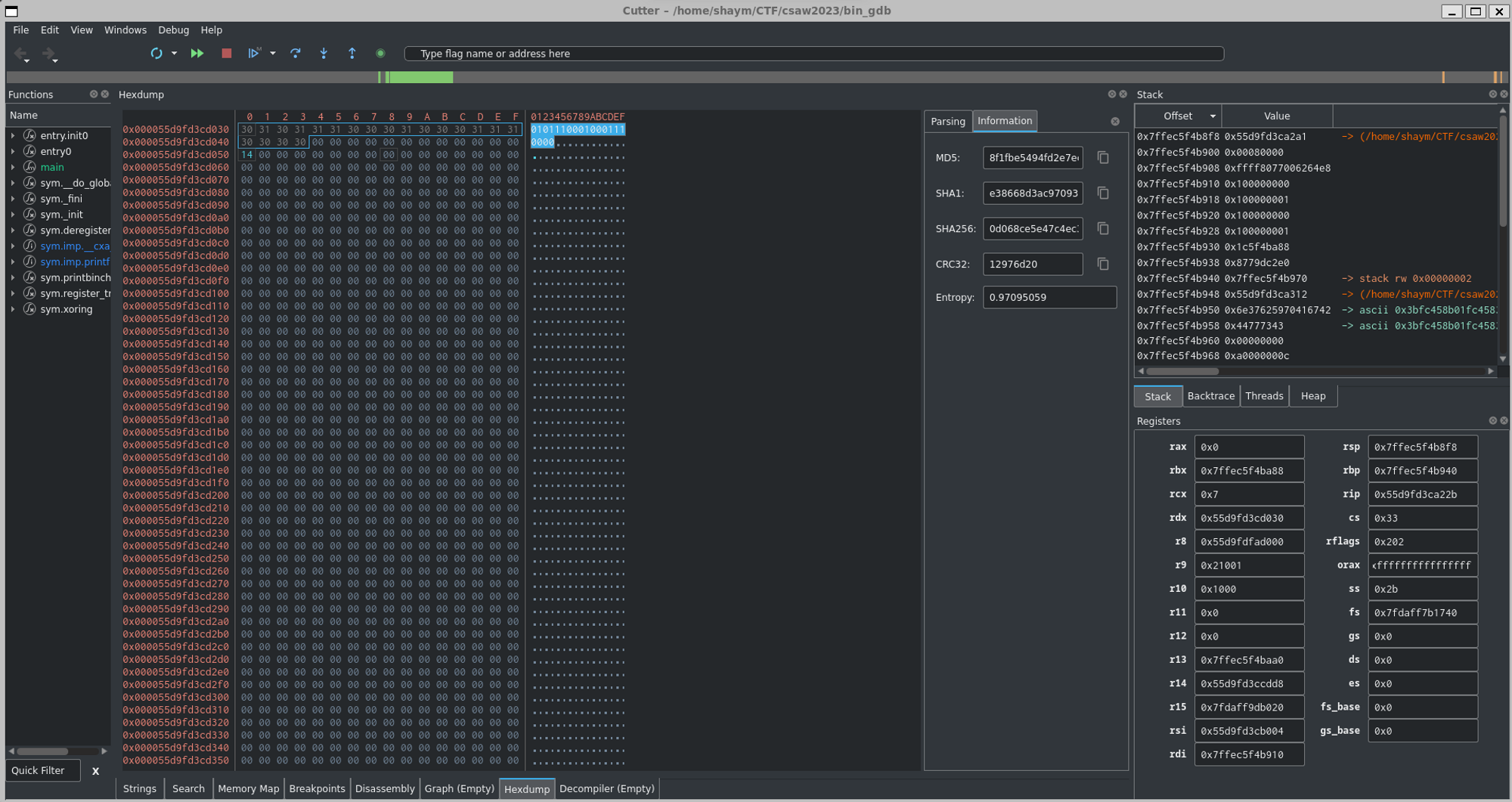
WriteUp made by Shaym